Classes and Methods
InputController
The InputController
class is a singleton component responsible for managing user input.
Key Public Fields, Properties, and Methods:
public int MaxTouchesCount {...}
- specifies the maximum number of touches allowed for simultaneous drawing.;public InputMethods ActiveInputMethods {...}
- defines active input methods: VRMode, Pen, Touch, Mouse. All input methods are enabled by default;public IList<Canvas> Canvases {...}
- holds a list of canvases that should ignore raycasts, preventing painting on their child objects;public bool BlockRaycastsOnPress {...}
- determines if painting should be blocked when hovering over canvas items;public GameObject[] IgnoreForRaycasts {...}
- GameObjects to ignore raycasts (children objects of Canvas that won't prevent painting);public Transform PenTransform
- Transform of Pen/Hand for painting, used for VR painting;public float MinimalDistanceToPaint
- minimal distance from Pen to surface to perform painting, used for VR painting;public event Action<int, Vector3> OnMouseHover
- triggered when the mouse hovers over an object;public event Action<int, Vector3, float> OnMouseDown
- triggered when a left mouse click occurs on an object;public event Action<int, Vector3, float> OnMouseButton
- triggered while the left mouse button is held down over an object;public event Action<int, Vector3> OnMouseUp
- triggered when the left mouse button is released over an object.
RaycastController
The RaycastController
class is a singleton responsible for handling ray intersection checks with triangles and storing data about all objects available for raycasting.
Key Public Fields, Properties, and Methods:
public bool UseDepthTexture {...}
- determines whether to use a Depth Texture for raycasting, useful when multiple PaintManagers are active in a scene. If using SRP, DepthTexture must be enabled in URP/HDRP settingspublic void InitObject(IPaintManager paintManager, Component paintComponent, Component renderComponent)
- initializes PaintManager object;public IList<Triangle> GetLineTriangles(IPaintManager paintManager, int fingerId)
- returns a list of triangles intersected by a line;public Mesh GetMesh(IPaintManager paintManager)
- returns the mesh associated with a PaintManager;public void DestroyMeshData(IPaintManager paintManager)
- destroys the mesh data created for raycasts;public void AddCallbackToRequest(IPaintManager sender, int fingerId, Action callback = null)
- adds a callback for the PaintManager request;public void RequestRaycast(IPaintManager sender, InputData inputData, InputData previousInputData, Action<RaycastRequestContainer> callback = null)
- creates a request to find ray-triangle intersections. This is performed in the LateUpdate method;public RaycastData TryGetRaycast(RaycastRequestContainer requestContainer, int fingerId, Vector3 pointerPosition)
- retrieves raycast data from a previously sent request;public RaycastData RaycastLocal(IPaintManager paintManager, Ray ray, Vector3 pointerPosition, int fingerId, bool useWorld = true)
- checks if a ray intersects any triangles and returns the corresponding raycast data.
PaintController
The PaintController
class is a singleton that manages all PaintManagers. It provides shared settings for all PaintManagers when the “Use Shared Settings” flag is enabled; otherwise, each PaintManager uses its own settings.
Key Public Fields, Properties, and Methods:
public bool OverrideCamera { … }
- determines if the camera used for raycasts should be overridden;public Camera Camera { … }
- reference to the camera used for raycasting;public bool UseSharedSettings
- specifies whether shared settings for brush, paint mode, and tool should be used across all PaintManagers;public PaintMode PaintMode { … }
- current painting mode;public PaintTool Tool { … }
- current tool used for painting;public Brush Brush { … }
- returns the brush instance;public void RegisterPaintManager(PaintManager paintManager)
- registers a PaintManager;public void UnRegisterPaintManager(PaintManager paintManager)
- unregisters a PaintManager;public IPaintMode GetPaintMode(PaintMode mode)
- returns an instance of the specified paint mode;public PaintManager[] GetActivePaintManagers()
- returns an array of active PaintManagers;public PaintManager[] GetAllPaintManagers()
- returns all registered PaintManagers;public IEnumerable<PaintManager> GetAllPaintManagersAsEnumerable()
- returns all registered PaintManagers as IEnumerable.
PaintManager
The PaintManager
class is the core component for managing painting operations on objects. It contains instances of the Paint class and the BasePaintObject.
Key Public Fields, Properties, and Methods:
public event Action<PaintManager> OnInitialized
- triggered when initialization completes; returns the PaintManager instance;public event Action OnDisposed
- triggered when the PaintManager resources are disposed;public GameObject ObjectForPainting { ... }
- the GameObject to be painted;public Paint Material { ... }
- the instance of the Paint class;public ILayersController LayersController {...}
- reference to LayersController instance;public LayersContainer LayersContainer {...}
- reference to LayersContainer instance;public FilterMode FilterMode { ... }
- defines the FilterMode of the RenderTextures;public IBrush Brush { ... }
- property of the brush used by PaintManager;public ToolsManager ToolsManager { ... }
- manages all painting tools within the PaintManager;public PaintTool Tool { ... }
- property of the current painting tool;public BasePaintObject PaintObject { … }
- property of the object being painted;public IStatesController StatesController { … }
- reference to the StatesController instance;public bool CopySourceTextureToLayer {...}
- indicates whether the source texture is copied to the layer;public bool UseSourceTextureAsBackground { … }
- indicates whether the source texture is used as a background texture;public bool HasTrianglesData { … }
- whether the component contains triangle data;public Triangles[] Triangles { … }
- get/set triangles array;public bool Initialized { … }
- indicates whether the object is initialized;public int SubMesh { … }
- defines the submesh of the paint object mesh;public int UVChannel { … }
- specifies the UV channel of the mesh;public PaintSpace PaintSpace {...}
- property of the selected paint space;public IPaintMode PaintMode
- returns the instance of the current paint mode;public void Init()
- initializes the PaintManager; if already initialized, it recreates internal data such as RenderTextures, meshes, and materials;public void DoDispose()
- destroys all resources (e.g., RenderTextures, meshes, materials) and restores the original material and texture;public bool IsActive()
- returns whether the PaintManager is currently able to perform painting;public void Render()
- triggers the rendering of the object;public void SetPaintMode(PaintMode paintMode)
- sets the current paint mode;public RenderTexture GetPaintTexture()
- returns the combined texture from the painting process;public RenderTexture GetPaintInputTexture()
- returns the input RenderTexture (current frame’s dot/line or input drawn between mouse events);public RenderTexture GetResultRenderTexture()
- returns the result RenderTexture (combined layers);public Texture2D GetResultTexture(bool hideBrushPreview = false)
- returns the final texture (combined layers); hiding the brush preview is optional; ensure proper disposal of the texture to avoid memory leaks;public SetLayersData(LayersContainer container)
- sets layer data;public LayerData[] GetLayersData()
- returns the current layer data;public bool IsLayersContainerExists(string filename)
- checks whether the LayersContainer exists on storage by filename;public bool SaveToLayersContainer(string filename)
- saves the LayersContainer to storage, returning true on success;public bool DeleteLayersContainer(string filename)
- deletes the LayersContainer from storage, returning true on success;public bool LoadFromLayersContainer(string filename)
- loads the LayersContainer from storage, returning true on success;public void InitBrush()
- initializes the brush settings;public Ray GetRay(Vector3 screenPosition)
- returns Ray from screen position.
BasePaintObject
The BasePaintObject
class is the foundation for painting on RenderTexture. Derived classes, such as CanvasRendererPaint
, SpriteRendererPaint
, MeshRendererPaint
, and SkinnedMeshRendererPaintObject
, implement logic for checking painting positions based on input data.
Key Public Fields, Properties, and Methods:
public event Action<PointerData> OnPointerHover
- mouse hover event. Arguments: PointerData that contains paint object local position, screen position, UV position, texture position, pressure, finger id;public event Action<PointerData> OnPointerDown
- mouse down event. Arguments: PointerData that contains paint object local position, screen position, UV position, texture position, pressure, finger id;public event Action<PointerData> OnPointerPress
- mouse press event. Arguments: PointerData that contains paint object local position, screen position, UV position, texture position, pressure, finger id;public event Action<PointerUpData> OnPointerUp
- mouse up event. Arguments: PointerUpData that contains screen position, is mouse in object bounds, finger id;public event Action<DrawPointData> OnDrawPoint
- draw point event, used by developers to obtain data about painting. Arguments: DrawPointData that contains the texture position, pressure, and finger ID;public event Action<DrawLineData> OnDrawLine
- draw line event, used by developers to obtain data about painting. Arguments: DrawLineData that contains the start and end texture positions, start and end pressure, and finger ID;public bool InBounds { … }
- property indicating whether the user’s object is within bounds for painting;public bool IsPainting { … }
- property indicating whether the user is actively painting;public bool IsPainted { … }
- property indicating whether painting has occurred in the current frame;public bool ProcessInput
- property indicating whether input processing is active for the current paint object;public void Init(IPaintManager paintManager, IPaintData paintData, Transform objectTransform, Paint paint)
- initializes the paint object;public void DoDispose()
- destroys previously created RenderTextures and meshes;public void OnMouseHover(InputData inputData, RaycastData raycastData)
- mouse hover method;public void OnMouseHoverFailed(InputData inputData)
- method invoked when a canvas item blocks the raycast during mouse hover;public void OnMouseDown(InputData inputData, RaycastData raycastData)
- mouse down method;public void OnMouseButton(InputData inputData, RaycastData raycastData)
- mouse button press method;public void OnMouseFailed(InputData inputData)
- method invoked when a canvas item blocks the raycast for mouse down/press events;public void OnMouseUp(InputData inputData)
- mouse up method;public Vector2? GetTexturePosition(InputData inputData, RaycastData raycastData)
- returns the paint position from the screen position if the ray intersects with an object;public FrameDataBuffer<FrameData> SavePaintState(int fingerId = 0)
- saves the paint state for the specified fingerId;public void RestorePaintState(FrameDataBuffer<FrameData> paintDataStorage, int fingerId = 0)
- restores the paint state for the specified fingerId;public void DrawPoint(DrawPointData drawPointData, Action onFinish = null)
- draws a point from code using DrawPointData;public void DrawPoint(InputData inputData, RaycastData raycastData, Action onFinish = null)
- draws a point from code using InputData and RaycastData;public void DrawLine(DrawLineData drawLineData, Action onFinish = null)
- draw line from code using DrawLineData;public void DrawLine(InputData inputDataStart, InputData inputDataEnd, RaycastData raycastDataStart, RaycastData raycastDataEnd, KeyValuePair<Ray, RaycastData>[] raycasts = null, Action onFinish = null)
- draw line from code using InputData and RaycastData;public void FinishPainting(int fingerId = 0, bool forceFinish = false)
- ends painting;public void RenderGeometries(bool finishPainting = false)
- render to combined RenderTexture;public void RenderToTextures()
- combines all RenderTextures into the final RenderTexture;public void RenderToTextureWithoutPreview(RenderTexture resultTexture)
- renders to the final RenderTexture without including the brush preview;public void SaveUndoTexture()
- saves the active layer texture to the Undo/Redo system;public void SetRaycastProcessor(BaseRaycastProcessor processor)
- sets the raycast processor.
StatesController
The StatesController
class manages the undo/redo states, handling changes to objects and their previous states.
Key Public Fields, Properties, and Methods:
public int ChangesCount { ... }
- the number of changes made;public event Action<RenderTexture> OnClearTextureAction
- event for clearing the RenderTexture;public event Action OnRenderTextureAction
- event for undo/redo operations, triggering the object to render;public event Action OnChangeState
- event for changing the state during undo/redo operations;public event Action OnResetState
- event to reset the state, typically used to clear the InputTexture;public event Action OnUndoRequested
- event triggered when an undo action is requested;public event Action OnUndo
- event triggered when an undo action is performed;public event Action OnRedoRequested
- event triggered when a redo action is requested;public event Action OnRedo
- event triggered when a redo action is performed;public event Action<bool> OnUndoStatusChanged
- event for monitoring changes to the undo status; returns a flag indicating whether an undo can be performed;public event Action<bool> OnRedoStatusChanged
- event for monitoring changes to the redo status; returns a flag indicating whether a redo can be performed;public void DoDispose()
- destroys the state data;public void Enable()
- enables undo/redo functionality;public void Disable()
- disables undo/redo functionality;public void AddState(Action action)
- adds a new state by specifying an action;public void AddState(object entity, string property, RenderTexture oldValue, RenderTexture newValue, Texture source)
- adds a RenderTexture change state;public void AddState(object entity, string property, object oldValue, object newValue)
- adds a property change state;public void AddState(IList collection, NotifyCollectionChangedEventArgs rawEventArg)
- adds a state to record changes to a list;public void Undo()
- performs an undo action;public void Redo()
- performs a redo action;public int GetUndoActionsCount()
- returns the number of available undo actions;public int GetRedoActionsCount()
- returns the number of available redo actions;public bool CanUndo()
- returns whether an undo action can be performed;public bool CanRedo()
- returns whether a redo action can be performed;public void EnableGrouping(bool useCustomGroup = false)
- enables grouping of actions; all actions will be recorded as one group until DisableGrouping() is invoked;public void DisableGrouping(bool useCustomGroup = false)
- disables action grouping.
LayersController
The LayersController
class manages layer instances and their properties, allowing the manipulation of layers within a painting context.
Key Public Fields, Properties, and Methods:
public event Action<ObservableCollection<ILayer>, NotifyCollectionChangedEventArgs> OnLayersCollectionChanged
- event triggered when the layers collection is changed;public event Action<ILayer> OnLayerChanged
- event triggered when a layer’s render properties change, used to trigger object rendering after layer parameters are modified;public event Action<ILayer> OnActiveLayerSwitched
- event to change active layer index;public event Action<bool> OnCanRemoveLayer
- event that returns a flag indicating whether a layer can be removed (at least one layer must remain for painting);public ReadOnlyCollection<ILayer> Layers { ... }
- collection of all layers;public ILayer ActiveLayer { ... }
- ithe currently active layer;public bool CanDisableLayer { ... }
- property indicating whether the active layer can be disabled (prevents disabling all layers);public bool CanRemoveLayer { ... }
- property indicating whether the active layer can be removed (prevents removing the last layer);public bool CanMergeLayers { ... }
- property indicating whether the active layer can be merged with the layer below it (both layers must be enabled);public bool CanMergeAllLayers { ... }
- property indicating whether all enabled layers can be merged (at least two layers must be enabled);public int ActiveLayerIndex { ... }
- index of the active layer;public void DoDispose()
- releases all layer data;public void Init(int width, int height)
- initializes the layers with the specified width and height;public void CreateBaseLayers(Texture sourceTexture, bool copySourceTextureToLayer, bool useSourceTextureAsBackground)
- creates default layers using the source texture;public void SetFilterMode(FilterMode mode)
- sets the filter mode for layer textures;public ILayer AddNewLayer()
- creates and adds a new layer;public ILayer AddNewLayer(string name)
- creates and adds a new layer with the specified name;public ILayer AddNewLayer(string name, Texture sourceTexture)
- creates and adds a new layer with the specified name and source texture;public void AddLayerMask(ILayer layer, Texture source)
- adds a layer mask using the specified source texture;public void AddLayerMask(ILayer layer)
- adds a layer mask to the specified layer;public void AddLayerMask(Texture source)
- adds a layer mask to the active layer using the source texture;public void AddLayerMask()
- adds a layer mask to the active layer;public void RemoveActiveLayerMask()
- removes the mask from the active layer;public ILayer GetActiveLayer()
- returns the active layer;public void SetActiveLayer(ILayer layer)
- sets the specified layer as active;public void SetActiveLayer(int index)
- sets the active layer by index;public void SetLayerOrder(ILayer layer, int index)
- sets the order of the specified layer;public void RemoveActiveLayer()
- removes the active layer;public void RemoveLayer(ILayer layer)
- removes the specified layer;public void RemoveLayer(int index)
- removes the layer by index;public void MergeLayers()
- merges the active layer with the layer below it (both must be enabled);public void MergeAllLayers()
- merges all enabled layers into the active layer (at least two layers must be enabled);public void SetLayerTexture(int index, Texture texture)
- sets the texture for the specified layer index;public Texture2D GetActiveLayerTexture()
- returns the texture of the active layer (Texture2D). Note that it should be destroyed properly to avoid memory leaks;public Texture2D GetLayerTexture(int layerIndex)
- returns the texture (Texture2D) of the layer at the specified index. Like GetActiveLayerTexture(), the texture should be disposed of properly.
Layer
The Layer
class represents an individual layer, managing its data and properties.
Key Public Fields, Properties, and Methods:
public event Action<ILayer> OnLayerChanged
- event triggered when layer properties are changed;public Action<Layer> OnRenderPropertyChanged
- event triggered when rendering properties that affect the layer are changed;public bool Enabled { ... }
- property indicating whether the layer is enabled;public bool CanBeDisabled { ... }
- property indicating whether the layer can be disabled;public bool MaskEnabled { ... }
- property indicating whether the mask is enabled for the layer;public string Name { ... }
- name of the layer;public float Opacity { ... }
- opacity of the layer;public Texture SourceTexture { ... }
- the source texture of the layer;public RenderTexture RenderTexture { ... }
- the render texture for the layer;public RenderTargetIdentifier RenderTarget { ... }
- identifier for the render texture;public Texture MaskSourceTexture { ... }
- the source texture for the mask;public RenderTexture MaskRenderTexture { ... }
- the render texture for the mask;public RenderTargetIdentifier MaskRenderTarget { ... }
- identifier for the mask render texture;public BlendingMode BlendingMode { ... }
- the blending mode of the layer;public void Create(string layerName, int width, int height, RenderTextureFormat format, FilterMode filterMode)
- creates a render texture for the layer with the specified parameters;public void Create(string layerName, Texture source, RenderTextureFormat format, FilterMode filterMode)
- creates a render texture for the layer using the specified source texture;public void Init(CommandBufferBuilder bufferBuilder, Func<bool> canDisableLayer)
- initializes the layer;public void AddMask(RenderTextureFormat format)
- creates a mask for the layer with the specified render texture format;public void AddMask(Texture maskTexture, RenderTextureFormat format)
- creates a mask for the layer using the specified texture and format;public void RemoveMask()
- removes the mask from the layer;public void SaveState()
- saves the state of the layer’s render texture using the StatesController;public void DoDispose()
- releases all layer data.
Paint
The Paint
class stores and manages painting material data and its parameters.
Key Public Fields, Properties, and Methods:
public Material PaintMaterial { … }
- the material used for painting;public Material PaintWorldMaterial { … }
- material used for painting in PaintSpace.World;public string ShaderTextureName { … }
- the name of the shader texture used for painting;public int DefaultTextureWidth { … }
- width of the texture if the object doesn’t have a source texture;public int DefaultTextureHeight { … }
- height of the texture if the object doesn’t have a source texture;public Color DefaultTextureColor { … }
- default color for the texture;public ProjectionMethod ProjectionMethod { … }
- determines the method used to project the brush texture onto the surface.
Usage:Planar Projection
: Projects the brush texture onto the surface along a single plane defined by the brush normal. Ideal for flat or slightly curved surfaces;Triplanar Projection
: Blends the brush texture across three orthogonal planes (X, Y, Z axes). Useful when the surface orientation varies significantly.
public ProjectionDirection ProjectionDirection { … }
- determines how the brush normal is calculated for texture projection.
Usage:Normal
: uses the triangle normal direction;Ray
: uses the raycast direction.
public float NormalCutout { … }
- parameter that ensures paint isn’t applied where the surface’s normal angle is too steep compared to the paint ray, helping avoid painting areas that face away from the painting ray in PaintSpace.World.
Usage:-1.0
: No culling based on normal direction (all surfaces are rendered);0.0
: Discards surfaces facing away from the viewer;1.0
: Only surfaces facing exactly towards the viewer are rendered.
public float ProjectionMaxAngle { … }
- specifies the maximum angle (in degrees) between the brush normal and the fragment normal for the brush to draw on surface. AdjustingMax Angle
allows you to control how much the brush wraps around curved surfaces in PaintSpace.World;public AngleAttenuationMode ProjectionAngleAttenuationMode { … }
- controls howMax Angle
affects the brush’s alpha value.
Usage:Smooth
Attenuation for natural, gradual blending on surfaces with varying orientations. Provides gradual transitions on curved surfaces;Hard
Cutoff for precise control, ensuring the brush effect is only applied within a specific angular range. The brush effect is fully applied to fragments within the angle threshold and completely absent beyond it.
public float ProjectionBrushDepth { … }
- controls the depth (thickness) of the brush effect along the brush normal direction, relative to the brush size. Useful for creating effects where the brush should only affect surfaces within a specific depth range in PaintSpace.World.
Usage:0.0
: Depth attenuation is disabled; the brush affects all fragments within its radius regardless of depth;> 0.0
: Limits the brush effect to a certain depth, creating a volumetric brush influence.
public float ProjectionDepthFadeRange { … }
- defines the range over which the brush effect fades out from full strength to zero along the depth specified byBrush Depth
. When greater than 0.0, it creates a smooth transition (fade) from full brush effect to no effect over the specified depth range in PaintSpace.World;public float ProjectionEdgeSoftness { … }
- controls the softness of the brush edges, affecting how the brush effect attenuates towards the outer radius in PaintSpace.World.
Usage:0.0
: Edge attenuation is disabled; the brush has a hard edge;> 0.0
: Applies an exponential falloff to the brush’s alpha value based on the radial distance from the center, resulting in softer edges.
public int MaterialIndex { … }
- index of the material within the object;public Texture SourceTexture { … }
- the source texture used by the paint material;public void Init(IRenderComponentsHelper renderComponents, Texture source)
- initializes the paint material using the render components and source texture;public void CreateWorldMaterial()
- creates a material for painting in PaintSpace.World;public void DoDispose()
- destroys previously created materials;public void RestoreTexture()
- restores the source texture to the material;public void SetObjectMaterialTexture(Texture texture)
- sets a new texture for the object;public void SetPreviewTexture(Texture texture)
- sets the texture used for previewing the paint;public void SetPaintTexture(Texture texture)
- sets the layer texture to the material;public void SetInputTexture(Texture texture)
- sets the input texture to the material;public void SetPreviewVector(Vector4 brushOffset)
- sets the vector data to display the brush preview.
Brush
The Brush
class stores and manages the brush material data and its parameters.
Key Public Fields, Properties, and Methods:
public event Action<Color> OnColorChanged
- event triggered when the brush color changes;public event Action<Texture> OnTextureChanged
- event triggered when the brush texture changes;public event Action<bool> OnPreviewChanged
- event triggered when the brush preview flag changes;public string Name { … }
- name of the brush, must be unique;public Material Material { … }
- material of the brush;public FilterMode FilterMode { … }
- filter mode of the brush’s RenderTexture;public Color Color { … }
- color of the brush;public Texture SourceTexture { … }
- source texture of the brush;public RenderTexture RenderTexture { … }
- RenderTexture of the brush;public float MinSize { … }
- minimum size of the brush;public float Size { … }
- size of the brush;public float RenderAngle { … }
- render angle of the brush in degrees;public Quaternion RenderQuaternion { … }
- render quaternion of the brush;public float Hardness { … }
- hardness of the brush;public bool Preview { … }
- flag indicating whether the brush preview is enabled;public void Init(IPaintMode mode)
- initializes the brush’s material, mesh, and RenderTexture;public void DoDispose()
- destroys the previously created RenderTexture, mesh, and material;public void SetValues(Brush brush)
- copies values from another brush;public void Render()
- renders the brush into the RenderTexture;public void RenderFromTexture(Texture texture)
- renders the brush from the texture without changing the SourceTexture;public void SetColor(Color colorValue, bool render = true, bool sendToEvent = true)
- sets the brush color and optionally renders it and sends the event;public void SetTexture(Texture texture, bool render = true, bool sendToEvent = true, bool canUpdateRenderTexture = true)
- sets the brush texture and optionally renders it, sends the event, and updates the RenderTexture.
ToolsManager
The ToolsManager
class manages all the tools available for painting within the PaintManager. It handles the registration and selection of painting tools.
Key Public Fields, Properties, and Methods:
public event Action<IPaintTool> OnToolChanged
- event triggered when the current tool is changed. Arguments: IPaintTool representing the new tool;public IPaintTool CurrentTool
- returns the current tool;public void Init(PaintManager paintManager)
- initializes the ToolsManager for the given PaintManager and subscribes to its events;public void DoDispose()
- releases all tool-related resources;public void SetTool(PaintTool paintTool)
- sets the specified tool as the active tool;public IPaintTool GetTool(PaintTool tool)
- retrieves the tool based on the PaintTool type.
BasePaintTool
The BasePaintTool
class is the base class for all painting tools. It processes layer textures using input events from the PaintManager.
Key Public Fields, Properties, and Methods:
public virtual PaintTool Type { … }
- type of the tool;protected IPaintData Data
- data that the tool has to process an image. Used to get information about layers, RenderTextures, Brush, painting states;public virtual bool AllowRender { … }
- indicates whether rendering is allowed;public virtual bool CanDrawLines { … }
- indicates whether the tool can draw lines. If false, the user can only draw dots;public virtual bool ConsiderPreviousPosition { … }
- determines whether the brush should render if the previous paint position hasn’t changed;public virtual bool ShowPreview { … }
- flag indicating whether to show the brush preview;public virtual int Smoothing { … }
- smoothing level for line drawing, values range from 1 to 10, where 1 disables smoothing and 10 provides maximum smoothness. Affects SpriteRenderer and RawImage components;public virtual bool RandomizePointsQuadsAngle { … }
- indicates whether to randomize angles of quads when drawing points;public virtual bool RandomizeLinesQuadsAngle { … }
- indicates whether to randomize angles of quads when drawing lines, useful for simulating spray tool behavior;public virtual bool RenderToLayer { … }
- indicates whether to render to the layer texture;public virtual bool RenderToInput { … }
- indicates whether to render to the input texture;public virtual bool RenderToTextures { … }
- indicates whether to render to any RenderTextures;public virtual bool DrawPreProcess { … }
- indicates whether to perform pre-processing before drawing;public virtual bool DrawProcess { … }
- indicates whether to perform drawing during the process;public virtual bool BakeInputToPaint { … }
- determines whether to bake the input texture to the layer texture;public virtual bool ProcessingFinished { … }
- returns whether the tool has finished processing;public virtual bool RequiredCombinedTempTexture { … }
- indicates whether the tool requires a temporary combined texture;public BasePaintToolSettings BaseSettings { … }
- reference to the base settings of the tool;public T Settings { … }
- reference to the specific settings class of the tool;public void FillWithColor(Color color)
- fills the active layer with the specified color;public virtual void OnDrawPreProcess(RenderTargetIdentifier combined)
- performs pre-processing before combining layers into the combined texture;public virtual void OnDrawProcess(RenderTargetIdentifier combined)
- dperforms drawing when combining layers into the combined texture.
BasePaintToolSettings
The BasePaintToolSettings
class is the base class for settings of all tools. Each tool can have its own derived settings class.
Key Public Fields, Properties, and Methods:
public bool CanPaintLines { … }
- indicates whether the tool can draw lines. If false, the user can only draw dots;public bool DrawOnBrushMoveOnly { … }
- determines whether the tool renders the brush only if the previous paint position has changed;public int Smoothing { … }
- defines the smoothing level for line drawing, values range from 1 to 10 (where 1 disables smoothing and 10 provides maximum smoothness). In the case of values greater than 1, line painting performs with a 2-frame delay. This parameter affects SpriteRenderer and RawImage components;public bool RandomizePointsQuadsAngle { … }
- indicates whether to randomize the angles of quads while drawing points;public bool RandomizeLinesQuadsAngle { … }
- indicates whether to randomize the angles of quads while drawing lines. It can be used to simulate spray tool behavior with an appropriate brush texture.
Tools parameters can be displayed in the Inspector tab if they have the [PaintToolSettings] attribute.
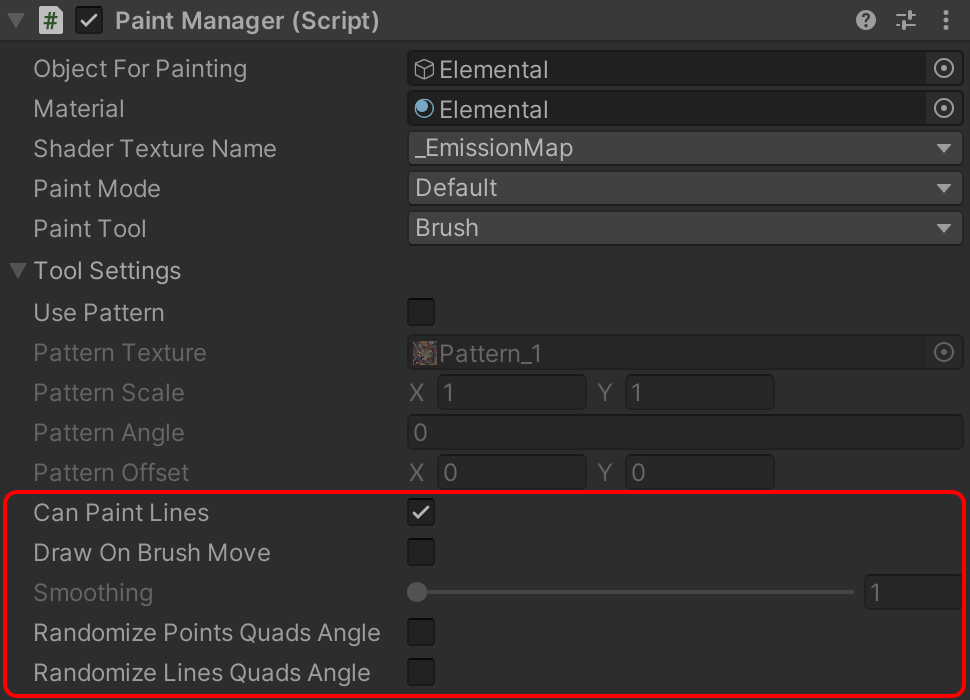
AverageColorCalculator
The AverageColorCalculator
component calculates the average color of a texture. It samples the texture based on set accuracy and can exclude transparent pixels if needed.
Key Public Fields, Properties, and Methods:
public event Action<Color> OnGetAverageColor
- event triggered when the average color is calculated during painting;public PaintManager PaintManager
- the PaintManager used to get the average color;public PaintRenderTexture PaintRenderTexture
- the texture on which the average color is calculated;public bool SkipTransparentPixels
- flag indicating whether to skip transparent pixels in the source texture during color sampling;public void SetAccuracy(int accuracy)
- sets the sampling accuracy for color calculation.
The AverageColorCalculator uses the shader XD Paint/Average Color to sample the texture and determine the average color. Two parameters control this process: the main texture and accuracy (which acts as a divider for the number of samples taken). Higher accuracy values increase the number of samples and GPU usage, while lower values improve performance but reduce accuracy.
ColliderPainter
The ColliderPainter
component allows painting on PaintObject surfaces using collision data. It captures raycast positions during OnCollisionEnter and OnCollisionStay events and uses the brush to paint on the object based on the collision data.
Key Public Fields, Properties, and Methods:
public public event Action<PaintManager>, Collision> OnCollide
- event triggered when a collision occurs, allowing painting based on the collision data. The first argument is the PaintManager, and the second is the collision data;public public Color Color
- color to use for painting during a collision;public public float Pressure
- value to scale the brush size during painting, simulating pressure sensitivity.