Quick Start Guide
Add a prefab to the scene from path Assets/XDPaint/Prefabs/[XDPaintContainer].prefab
. This prefab contains singleton components required for work of the asset.
Input Controller
This singleton class handles the management of user input. Let’s look at the parameters of the InputController component:
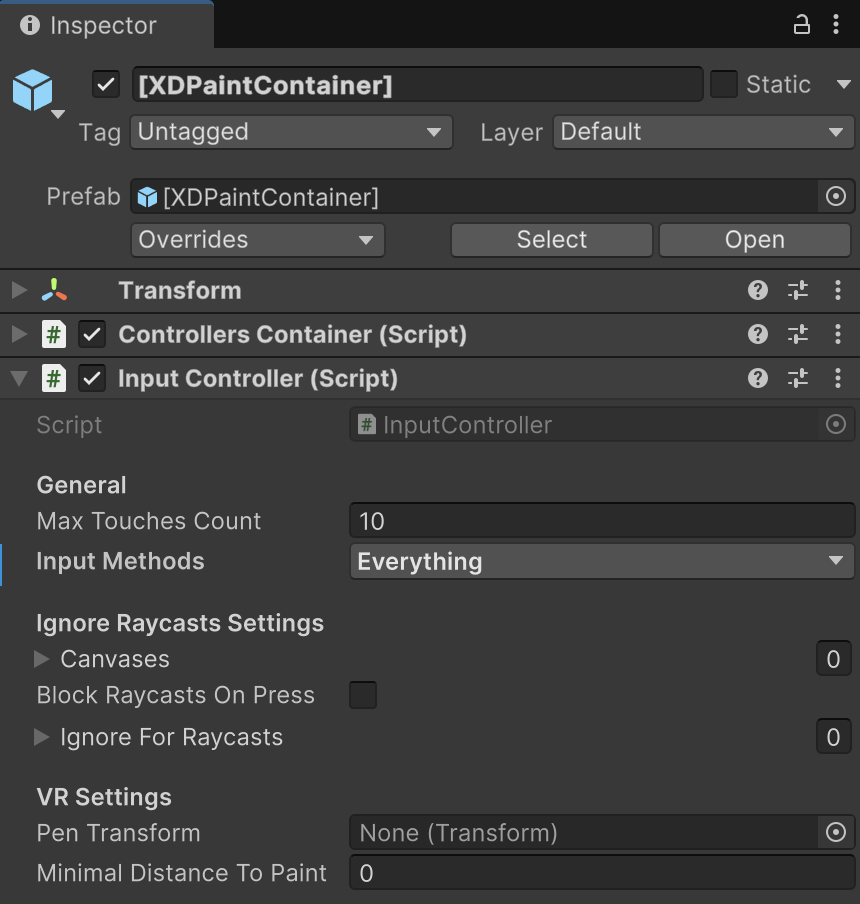
Max Touches Count
- maximum number of touches for simultaneous drawing;Input Methods
- active input methods: VRMode, Pen, Touch, Mouse. By default, all input methods are active;Canvases
- Canvas list to ignore raycasts (children objects of Canvas will prevent painting);Block Raycasts On Press
- whether to block painting when user hover the canvas items or not;Ignore For Raycasts
- GameObjects to ignore raycasts (children objects of Canvas that won't prevent painting);Pen Transform
- Transform of Pen/Hand for painting, used for VR device;Minimal Distance To Paint
- minimal distance from Pen to surface to perform painting, used for VR painting.
Raycast Controller
This singleton class manages data checks for ray intersections with triangles and stores information about all available objects for performing raycast checks. Let’s look at the parameters of the RaycastController component:
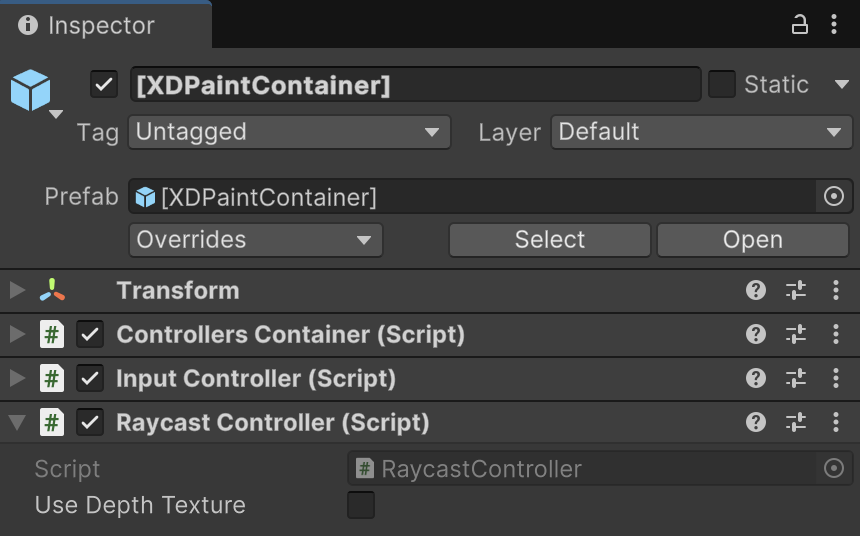
Use Depth Texture
- whether to use Depth Texture for checking raycasts. This is useful in cases when more than one PaintManager is active on the scene, because in this case, it will paint on the nearest PaintManager to the ray origin. Note that if you use SRP, you need to enable DepthTexture in the URP/HDRP settings.
Paint Controller
This singleton class stores all PaintManagers. Let’s look at the parameters of the PaintController component:
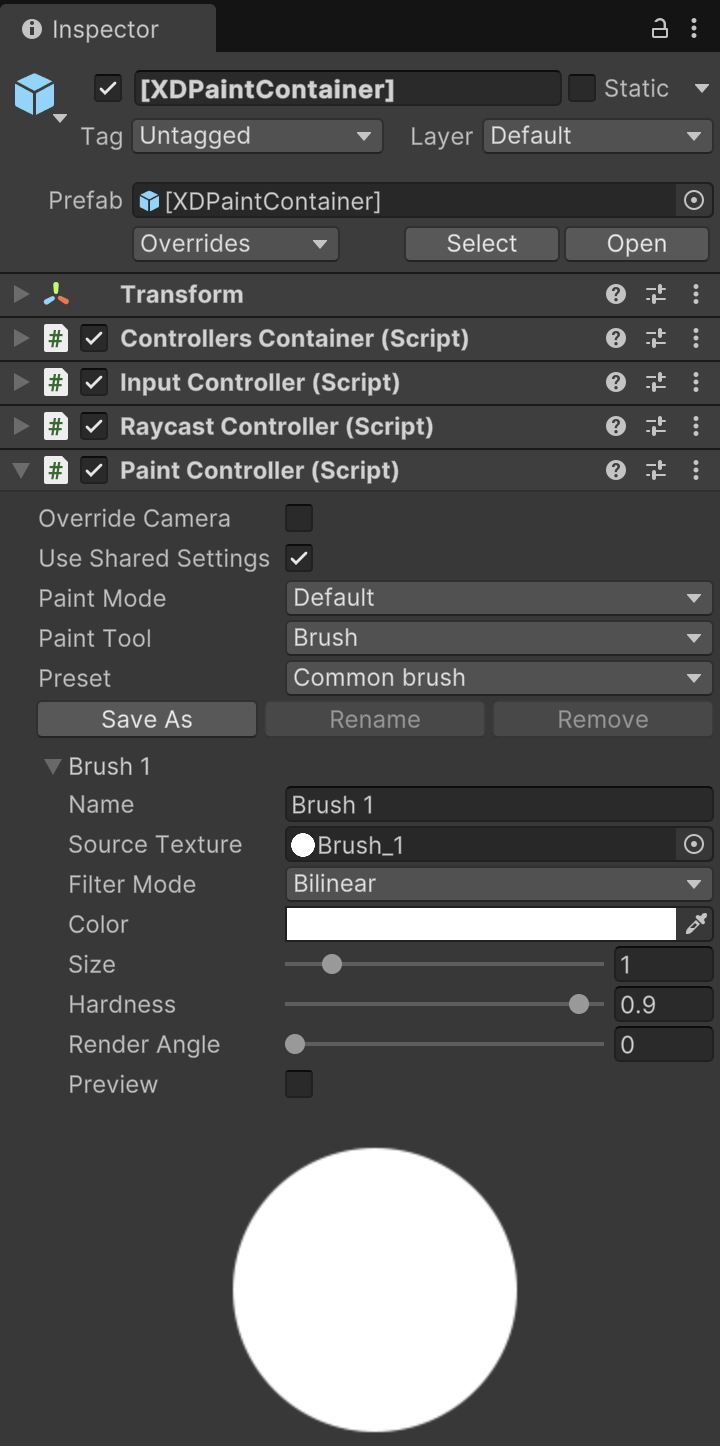
Override Camera
- set the camera for the InputController and RaycastController objects, singleton objects. If unchecked, Camera.main will be used;Use Shared Settings
- whether to use settings of PaintController (Brush, Paint Tool, and Paint Mode) for all PaintManager instances. If flag is disabled each PaintManager uses its own individual settings;Paint Mode
- the painting mode can be set to either Default or Additive. In Default mode, the drawing results are baked into the Layer Texture each frame. In Additive mode, more precise color and alpha blending is achieved by rendering lines onto the Input Texture, with the results baked into the Layer Texture upon the Mouse Up event;Paint Tool
- selected tool. Supported tools:Brush
- brush tool for painting on the texture;Erase
- erase tool;Bucket
- tool for filling texture areas with the selected color;Eyedropper
- eyedropper tool for picking the color of the brush;BrushSampler
- tool for sampling brush texture;Clone
- tool for cloning parts of the texture;Blur
- tool for blurring parts of the texture;GaussianBlur
- tool for blurring parts of the texture;Grayscale
- tool for discolorating parts of the texture.
Preset
- brush preset saved inAssets/XDPaint/Resources/XDPaintBrushPresets.asset
. It stores brushes with different parameters to easily switch between them. See Brush and Presets paragraph for details.
Brush Parameters:
Name
- name of the brush;Source Texture
- brush texture. When you add a new brush, make sure that «Wrap Mode» is set as «Clamp» in the settings of the brush texture;Filter Mode
- FilterMode for brush RenderTexture;Color
- brush color;Size
- brush size;Hardness
- brush hardness. Rounds brush when a value is less than 1;Render Angle
- brush render angle in degrees;Preview
- to show a preview of the brush while the user hovers over the object to be painted;
Paint Manager
This class manages the paint object, serving as the core component for painting on objects.
Create the GameObject with the PaintManager
component using the Unity menu «GameObject -> 2D/3D Paint», or add the PaintManager
component to your GameObject. Then select the GameObject to paint in the Object For Painting
field. The object to paint must contain one of the supported components: MeshRenderer
, SkinnedMeshRenderer
, SpriteRenderer
or RawImage
. If any child of PaintManager
GameObject contains an object with one of the supported components, it can automatically assign it to the Object For Painting
field by clicking on the Auto Fill
button:
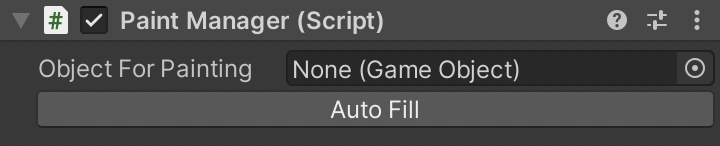
After clicking on the Auto Fill
button, the Object For Painting field will be filled in when one of the supported components is found:
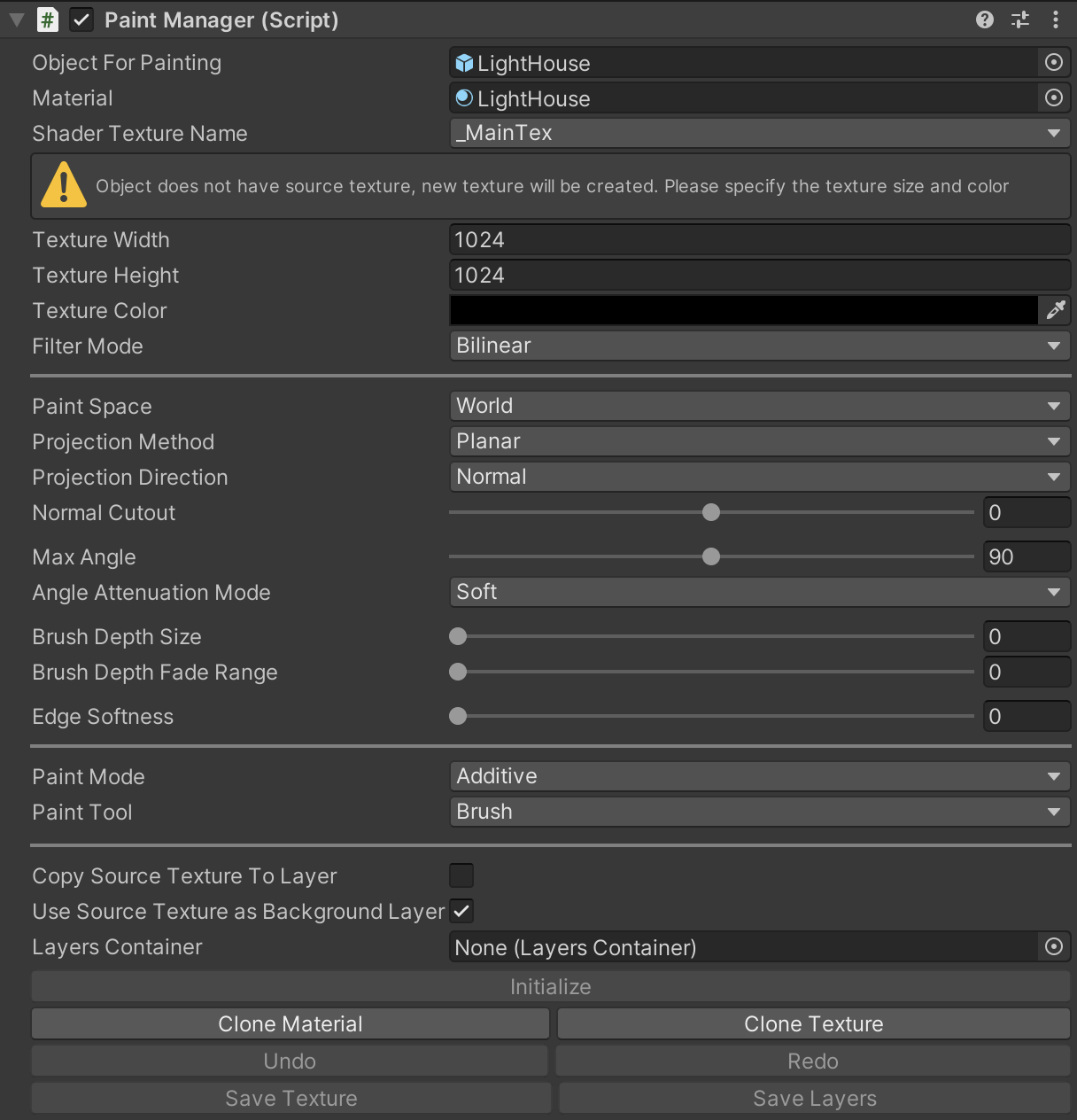
If the material or paint object cannot be found automatically, make sure that there is a GameObject with the supported component among the child objects, otherwise, Object For Painting and Material can be assigned manually.
Other component settings will appear if Object For Painting and Material have values. Consider the existing settings:
Object For Painting
- GameObject for painting, must contain one of the supported components;Material
- the material of the object whose texture field (Shader Texture Name) will be used for drawing;Shader Texture Name
- the name of the material texture on which it will perform the painting;Texture Width
- the default texture width is used for objects that do not have the source texture;Texture Height
- the default texture height is used for objects that do not have the source texture;Texture Color
- the pixels color of the texture that will be created in case of source texture is not specified;Filter Mode
- FilterMode for painting RenderTextures;UV Channel
- the UV channel;Paint Space
- specifies the coordinate space used for projecting the brush texture onto the surface:UV
: Projects the brush texture using the object’s UV coordinates;World
: Projects brush texture based on world positions for seamless painting across surfaces.
Projection Method
- determines the method used to project the brush texture onto the surface;Projection Direction
- determines how the brush normal is calculated for texture projection;Normal Cutout
- controls the cutoff threshold based on the angle between the surface normal and the viewing direction. By setting a threshold, it helps avoid painting on parts of the model that face away from the painting ray or camera, like the back faces, when working in World Paint Space;Max Angle
- specifies the maximum angle (in degrees) between the brush normal and the fragment normal for the brush to draw on surface. AdjustingMax Angle
allows you to control how much the brush wraps around curved surfaces;Angle Attenuation Mode
- controls howMax Angle
affects the brush’s alpha value;Brush Depth Size
- controls the depth (thickness) of the brush effect along the brush normal direction, relative to the brush size. Useful for creating effects where the brush should only affect surfaces within a specific depth range;Brush Depth Fade Range
- defines the range over which the brush effect fades out from full strength to zero along the depth specified byBrush Depth
. When greater than 0.0, it creates a smooth transition (fade) from full brush effect to no effect over the specified depth range;Edge Softness
- controls the softness of the brush edges, affecting how the brush effect attenuates towards the outer radius;Paint Mode
- mode of painting: default or additive;Paint Tool
- painting tool, if PaintController has checked Use Shared Settings flag, the tool from PaintController will be used as Paint Tool for all PaintManagers;Brush
- brush parameters. When selected «Custom» - PaintManager has unique brush parameters. You can select a ready-to-use preset of the brush from the list. Note that if PaintController has the flag Use Shared Settings, brush parameters will be used from PaintController;Copy Source Texture To Layer
- copy source texture from material to new layer;Use Source Texture as Background Layer
- copy source texture from material to background layer;Layers Container
- a reference to the ScriptableObject of LayersContainer, that contains information about layers, their textures, and parameters. Used for loading previously saved layers.Clone Material
- button to copy the source material of the object to the new file, can be used only in the Unity Editor;Clone Texture
- button to copy the source texture of the object to the new file, can be used only in the Unity Editor;Undo
- button to undo action with the object;Redo
- button to redo action with the object;Bake
- button to save the painting results to the source file. Note that texture is stored in RAM and is not written on the disk;Save Texture
- button to save the texture of the painting results to the file, can only be used in the Unity Editor;Save Layers
- button to save layers data to ScriptableObject. Let's set up the object to paint on the emission texture:

The texture of shader with the name _EmissionMap
is selected. After switching to the game mode, the user will be able to paint on the object LightHouse
. The material and the source texture _EmissionMap
will be cloned, RenderTexture will be assigned as the new texture. Painting can be done with various input devices, such as a mouse, touch screen, tablet stylus, or VR controller.